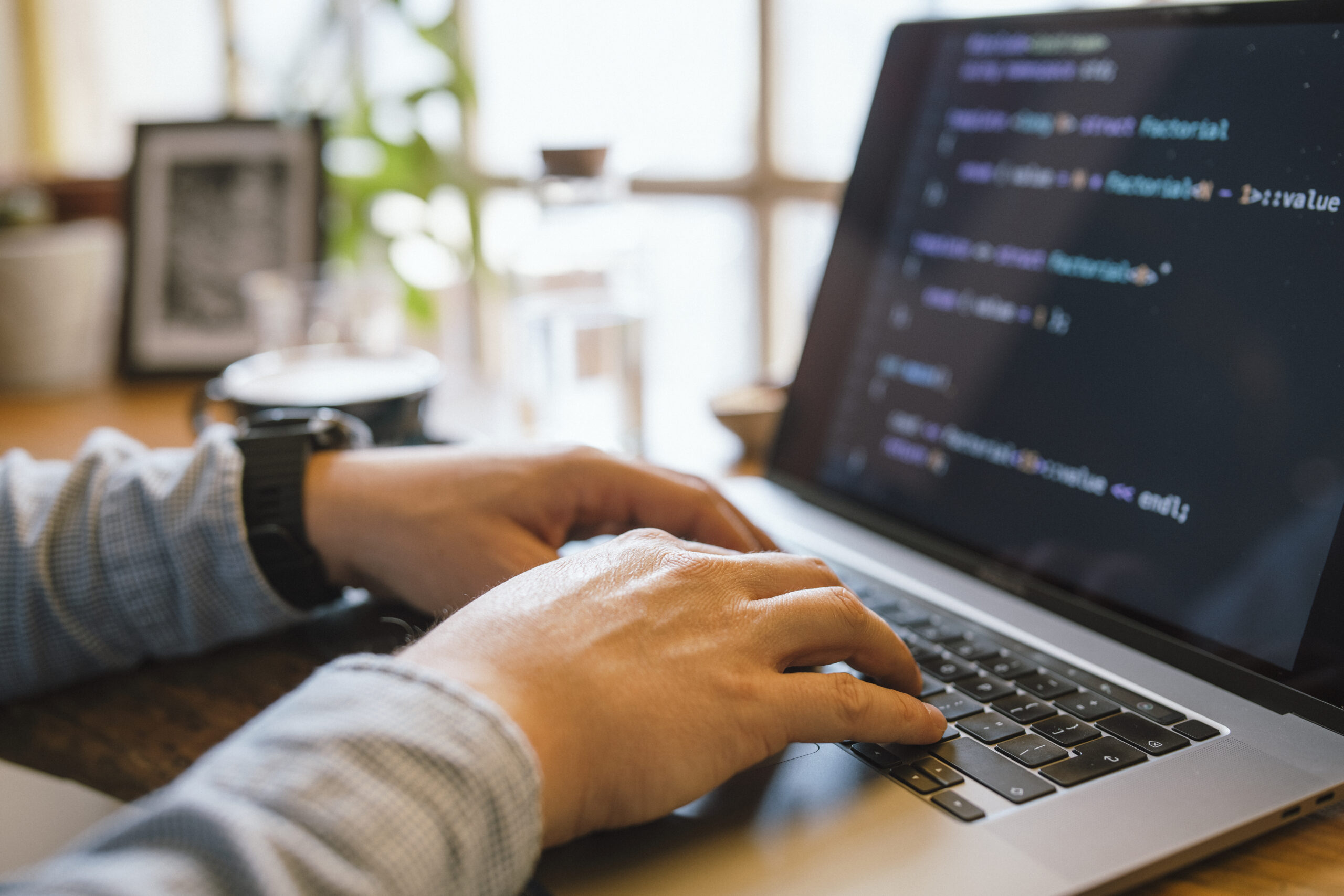
Debugging is Just about the most critical — however usually neglected — expertise in the developer’s toolkit. It's not almost correcting damaged code; it’s about comprehending how and why factors go Incorrect, and Mastering to Believe methodically to solve issues successfully. Whether or not you're a novice or possibly a seasoned developer, sharpening your debugging techniques can help you save several hours of irritation and radically help your efficiency. Here i will discuss quite a few tactics that can help builders stage up their debugging match by me, Gustavo Woltmann.
Grasp Your Resources
One of many fastest techniques developers can elevate their debugging competencies is by mastering the applications they use on a daily basis. Even though creating code is 1 part of development, recognizing tips on how to communicate with it successfully all through execution is Similarly essential. Fashionable progress environments arrive Geared up with strong debugging capabilities — but lots of developers only scratch the surface of what these instruments can do.
Choose, by way of example, an Integrated Progress Surroundings (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These equipment permit you to established breakpoints, inspect the value of variables at runtime, step by way of code line by line, as well as modify code over the fly. When employed correctly, they Enable you to notice just how your code behaves during execution, that's invaluable for tracking down elusive bugs.
Browser developer applications, including Chrome DevTools, are indispensable for entrance-finish builders. They allow you to inspect the DOM, keep an eye on community requests, check out authentic-time overall performance metrics, and debug JavaScript from the browser. Mastering the console, sources, and network tabs can switch frustrating UI concerns into workable responsibilities.
For backend or program-stage builders, tools like GDB (GNU Debugger), Valgrind, or LLDB offer you deep Command more than managing procedures and memory administration. Studying these equipment can have a steeper Studying curve but pays off when debugging functionality difficulties, memory leaks, or segmentation faults.
Beyond your IDE or debugger, grow to be relaxed with Variation Command devices like Git to understand code background, locate the exact minute bugs ended up introduced, and isolate problematic modifications.
In the end, mastering your equipment signifies heading outside of default configurations and shortcuts — it’s about developing an intimate knowledge of your improvement atmosphere in order that when troubles occur, you’re not dropped at nighttime. The higher you recognize your equipment, the more time you'll be able to devote fixing the actual issue instead of fumbling via the process.
Reproduce the Problem
Probably the most critical — and infrequently missed — ways in productive debugging is reproducing the situation. Ahead of jumping into the code or making guesses, builders need to have to make a constant environment or state of affairs the place the bug reliably appears. Without reproducibility, correcting a bug gets a sport of chance, normally resulting in wasted time and fragile code variations.
Step one in reproducing an issue is accumulating as much context as you possibly can. Ask issues like: What actions triggered The problem? Which environment was it in — progress, staging, or creation? Are there any logs, screenshots, or error messages? The greater depth you have, the less complicated it gets to be to isolate the precise situations less than which the bug happens.
Once you’ve collected more than enough data, try to recreate the situation in your local natural environment. This could indicate inputting exactly the same facts, simulating comparable consumer interactions, or mimicking method states. If The difficulty appears intermittently, take into account crafting automated assessments that replicate the sting circumstances or condition transitions associated. These exams not simply help expose the challenge but will also avoid regressions Sooner or later.
Sometimes, the issue can be environment-certain — it would materialize only on particular working devices, browsers, or less than specific configurations. Employing instruments like Digital machines, containerization (e.g., Docker), or cross-browser screening platforms might be instrumental in replicating these types of bugs.
Reproducing the problem isn’t just a phase — it’s a frame of mind. It necessitates tolerance, observation, along with a methodical strategy. But as soon as you can continually recreate the bug, you might be now midway to correcting it. With a reproducible scenario, You should use your debugging resources a lot more properly, examination likely fixes safely and securely, and communicate more clearly with your team or users. It turns an abstract criticism right into a concrete obstacle — Which’s wherever builders thrive.
Study and Comprehend the Error Messages
Mistake messages will often be the most beneficial clues a developer has when a little something goes Completely wrong. In lieu of observing them as aggravating interruptions, developers should learn to take care of mistake messages as direct communications from the procedure. They generally inform you just what happened, where by it took place, and at times even why it happened — if you know the way to interpret them.
Start off by looking through the message carefully As well as in total. Many builders, especially when less than time force, glance at the main line and quickly commence earning assumptions. But further in the mistake stack or logs might lie the legitimate root lead to. Don’t just copy and paste mistake messages into search engines like yahoo — browse and recognize them initial.
Split the error down into areas. Is it a syntax error, a runtime exception, or a logic error? Will it level to a selected file and line variety? What module or function activated it? These questions can information your investigation and stage you towards the liable code.
It’s also beneficial to understand the terminology of your programming language or framework you’re making use of. Mistake messages in languages like Python, JavaScript, or Java often comply with predictable styles, and Understanding to acknowledge these can drastically accelerate your debugging system.
Some mistakes are obscure or generic, As well as in These situations, it’s crucial to examine the context through which the mistake occurred. Check out similar log entries, input values, and recent alterations in the codebase.
Don’t forget about compiler or linter warnings both. These normally precede bigger concerns and supply hints about opportunity bugs.
Ultimately, error messages will not be your enemies—they’re your guides. Mastering to interpret them correctly turns chaos into clarity, assisting you pinpoint troubles speedier, cut down debugging time, and become a a lot more successful and self-assured developer.
Use Logging Sensibly
Logging is one of the most potent resources within a developer’s debugging toolkit. When utilised proficiently, it offers authentic-time insights into how an software behaves, serving to you fully grasp what’s occurring beneath the hood while not having to pause execution or action from the code line by line.
A superb logging system starts off with figuring out what to log and at what stage. Prevalent logging stages incorporate DEBUG, Data, WARN, ERROR, and Lethal. Use DEBUG for in-depth diagnostic information and facts through progress, Data for basic activities (like effective start-ups), Alert for probable troubles that don’t break the application, Mistake for true issues, and FATAL if the technique can’t carry on.
Keep away from flooding your logs with extreme or irrelevant information. An excessive amount of logging can obscure vital messages and decelerate your method. Focus on vital gatherings, condition changes, enter/output values, and demanding conclusion factors in your code.
Structure your log messages Obviously and continuously. Incorporate context, like timestamps, ask for IDs, and function names, so it’s much easier to trace concerns in dispersed techniques or multi-threaded environments. Structured logging (e.g., JSON logs) will make it even simpler to parse and filter logs programmatically.
During debugging, logs Enable you to track how variables evolve, what problems are met, and what branches of logic are executed—all with no halting the program. They’re Specially beneficial in generation environments exactly where stepping by code isn’t feasible.
Also, use logging frameworks and tools (like Log4j, Winston, or Python’s logging module) that assist log rotation, filtering, and integration with checking dashboards.
In the end, clever logging is about stability and clarity. That has a well-imagined-out logging solution, you'll be able to lessen the time it takes to spot troubles, gain deeper visibility into your programs, and Increase the overall maintainability and reliability of the code.
Imagine Like a Detective
Debugging is not only a complex undertaking—it is a form of investigation. To efficiently establish and take care of bugs, developers should technique the method similar to a detective resolving a get more info secret. This state of mind aids break down intricate difficulties into workable parts and follow clues logically to uncover the root result in.
Start off by accumulating proof. Think about the indications of the condition: mistake messages, incorrect output, or performance problems. Much like a detective surveys a crime scene, gather as much appropriate facts as you could without the need of leaping to conclusions. Use logs, exam conditions, and person experiences to piece alongside one another a transparent photo of what’s taking place.
Up coming, type hypotheses. Inquire your self: What could be causing this actions? Have any variations not long ago been designed on the codebase? Has this concern occurred before less than very similar conditions? The aim is always to narrow down alternatives and establish prospective culprits.
Then, check your theories systematically. Try to recreate the condition in the controlled ecosystem. When you suspect a particular function or part, isolate it and verify if The difficulty persists. Just like a detective conducting interviews, inquire your code thoughts and Allow the results guide you closer to the reality.
Pay out shut consideration to little aspects. Bugs typically hide from the least predicted locations—similar to a missing semicolon, an off-by-a person error, or simply a race problem. Be complete and client, resisting the urge to patch the issue with no totally knowledge it. Short term fixes may cover the actual difficulty, just for it to resurface later.
And lastly, maintain notes on That which you attempted and uncovered. Just as detectives log their investigations, documenting your debugging course of action can save time for long run problems and enable Other people recognize your reasoning.
By thinking just like a detective, builders can sharpen their analytical skills, strategy challenges methodically, and become simpler at uncovering concealed challenges in complicated programs.
Generate Tests
Creating exams is among the best tips on how to improve your debugging competencies and overall improvement effectiveness. Assessments not simply enable capture bugs early but will also serve as a safety net that gives you self-assurance when generating improvements on your codebase. A perfectly-analyzed software is much easier to debug mainly because it helps you to pinpoint exactly where and when a problem occurs.
Start with device checks, which deal with unique capabilities or modules. These compact, isolated checks can promptly expose no matter if a certain piece of logic is Functioning as anticipated. When a test fails, you immediately know where by to glimpse, noticeably cutting down enough time put in debugging. Unit checks are Primarily handy for catching regression bugs—troubles that reappear right after Formerly being preset.
Following, integrate integration tests and close-to-conclusion exams into your workflow. These assist ensure that several areas of your application do the job jointly easily. They’re significantly handy for catching bugs that take place in complex devices with several components or expert services interacting. If one thing breaks, your checks can inform you which part of the pipeline unsuccessful and below what ailments.
Creating checks also forces you to think critically about your code. To check a characteristic properly, you may need to know its inputs, predicted outputs, and edge cases. This standard of comprehending Obviously leads to higher code composition and fewer bugs.
When debugging a concern, writing a failing examination that reproduces the bug is usually a powerful initial step. As soon as the check fails continually, you can center on correcting the bug and observe your take a look at go when the issue is solved. This solution ensures that the identical bug doesn’t return Down the road.
In short, creating assessments turns debugging from the disheartening guessing sport into a structured and predictable course of action—assisting you catch additional bugs, a lot quicker and much more reliably.
Get Breaks
When debugging a difficult challenge, it’s quick to become immersed in the issue—watching your display screen for hrs, hoping Alternative after Answer. But Just about the most underrated debugging equipment is actually stepping away. Using breaks will help you reset your head, lower annoyance, and infrequently see The difficulty from the new point of view.
When you are way too near to the code for also prolonged, cognitive tiredness sets in. You could commence overlooking apparent mistakes or misreading code which you wrote just hrs earlier. Within this state, your Mind will become a lot less successful at dilemma-solving. A short wander, a espresso split, or perhaps switching to a different endeavor for ten–15 minutes can refresh your target. Numerous builders report acquiring the basis of an issue after they've taken the perfect time to disconnect, allowing their subconscious function in the history.
Breaks also support avoid burnout, Particularly all through extended debugging periods. Sitting before a screen, mentally trapped, is not merely unproductive but additionally draining. Stepping absent lets you return with renewed Power in addition to a clearer frame of mind. You may instantly observe a missing semicolon, a logic flaw, or maybe a misplaced variable that eluded you just before.
If you’re caught, a good guideline would be to established a timer—debug actively for 45–60 minutes, then have a 5–ten minute crack. Use that time to maneuver around, stretch, or do a little something unrelated to code. It might experience counterintuitive, Specially less than restricted deadlines, but it really truly causes more quickly and more practical debugging In the end.
Briefly, having breaks just isn't an indication of weakness—it’s a smart tactic. It gives your brain Room to breathe, increases your perspective, and will help you steer clear of the tunnel vision that often blocks your development. Debugging is usually a mental puzzle, and rest is a component of resolving it.
Learn From Each and every Bug
Just about every bug you encounter is more than just A brief setback—It can be an opportunity to develop being a developer. Irrespective of whether it’s a syntax error, a logic flaw, or perhaps a deep architectural concern, each can train you a little something beneficial should you make time to replicate and analyze what went Improper.
Start off by inquiring on your own some vital questions once the bug is resolved: What brought on it? Why did it go unnoticed? Could it have already been caught previously with greater techniques like device screening, code testimonials, or logging? The solutions typically reveal blind spots inside your workflow or knowing and enable you to Construct more powerful coding behavior shifting forward.
Documenting bugs can also be an excellent habit. Continue to keep a developer journal or manage a log in which you Observe down bugs you’ve encountered, the way you solved them, and Whatever you realized. With time, you’ll start to see styles—recurring difficulties or widespread problems—which you can proactively steer clear of.
In team environments, sharing Anything you've figured out from a bug together with your friends might be Specifically potent. Whether it’s via a Slack concept, a brief produce-up, or a quick knowledge-sharing session, serving to Other folks avoid the similar concern boosts team performance and cultivates a more powerful Discovering lifestyle.
Much more importantly, viewing bugs as classes shifts your attitude from frustration to curiosity. In place of dreading bugs, you’ll begin appreciating them as critical areas of your development journey. In spite of everything, a few of the most effective developers are certainly not the ones who produce ideal code, but people that constantly study from their errors.
Eventually, Each and every bug you take care of adds a whole new layer to your ability established. So next time you squash a bug, take a minute to replicate—you’ll arrive absent a smarter, extra capable developer as a consequence of it.
Summary
Enhancing your debugging techniques takes time, follow, and endurance — but the payoff is big. It would make you a far more effective, self-confident, and able developer. Another time you're knee-deep within a mysterious bug, recall: debugging isn’t a chore — it’s a possibility to become much better at Whatever you do.